이번 프로젝트에서는 SMTP 메일 송신 기능 구현이 필요했다. 3년 전 프로젝트에서 PDF를 만들고 그 파일을 메일에 첨부해 송신하는 요구사항이 있어서 구현했었는데 소스를 보관하지 않아 다시 만들어 봤다.
별 특별한 것은 없지만 회사내 개발자 PC와 메일시스템 포트를 막아버려 승인된 서버에서만 SMTP 발송이 가능하게 해놓았으므로 네이버 계정으로 개발을 했다. 구글도 가능하겠지.
프론트에서 Controller를 호출. 메일 발신 기능을 이용할 경우는 파일 첨부 방식이 약간 다를 것이다. 난 서버에 파일을 특정위치에 올려 두고 File 객체를 사용한다.
훗날 타 프로젝트 수행 시 같은 요구사항 들어오면 소스만 복붙해서 끝낼 마음으로 포스팅한다.
웹어플리케이션 서버, 자바버전 마다 mail.jar 문제가 있을 수 있지만 소스의 객체들은 비슷비슷하니 그리 난이도가 높지는 않다.
1. 메일 인증
public class MailAuth extends Authenticator{
PasswordAuthentication pa;
public MailAuth() {
String mail_id = "xxxx"; //네이버 아이디
String mail_pw = "xxxxx"; //네이버 비번
pa = new PasswordAuthentication(mail_id, mail_pw);
}
public PasswordAuthentication getPasswordAuthentication() {
return pa;
}
}
2. 파일 첨부 메일송신 메서드
public void sendMessage(String[] recipient_to,
String[] recipient_cc,
String[] pathFileInfo,
String subject,
String body,
String from,
String smtp_host_name,
String smtp_port) throws MessagingException {
SimpleDateFormat formatter = new SimpleDateFormat("yyyy년 MM월 dd일 hh시 mm분 ss초");
String sysdate = formatter.format(new Date());
Properties props = new Properties();
/**네이버로 테스트
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.naver.com");
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.port", "587");
**/
props.put("mail.smtp.host", smtp_host_name);
props.put("mail.smtp.port", smtp_port);
//MailAuth auth = new MailAuth(); 익명으로
//Session session = Session.getInstance(props, auth); 익명으로
Session session = Session.getInstance(props);
Message message = new MimeMessage(session);
InternetAddress addressFrom = new InternetAddress(from);
message.setFrom(addressFrom); //보내는 이
message.setSubject(subject); //제목
message.setSentDate(new Date()); //시간
InternetAddress[] addressTo = new InternetAddress[recipient_to.length];
for (int i = 0; i < recipient_to.length; i++) {
addressTo[i] = new InternetAddress(recipient_to[i]);
}
message.setRecipients(Message.RecipientType.TO, addressTo);
// 메일 콘텐츠 설정
Multipart mParts = new MimeMultipart();
MimeBodyPart mTextPart = new MimeBodyPart();
// 메일 콘텐츠 - 내용
/**********************************************
* 본문 처리
**********************************************/
mTextPart.setText(body, "UTF-8", "html");
mParts.addBodyPart(mTextPart);
/**********************************************
* 파일 처리. 첨부수대로 처리
**********************************************/
int cnt = pathFileInfo.length;
for(int i=0; i < cnt ;i++) {
MimeBodyPart attachPart = new MimeBodyPart();
DataSource source = new FileDataSource(pathFileInfo[i]);
attachPart.setDataHandler(new DataHandler(source));
//파일명칭이 깨지지 않도록 조치를 취함
try {
attachPart.setFileName(MimeUtility.encodeText(source.getName(), "euc-kr","B"));
mParts.addBodyPart(attachPart);
} catch (UnsupportedEncodingException e) {
System.out.println("파일 endcode 에러 발생");
System.out.println(e.getMessage());
e.printStackTrace();
}
}
/**********************************************
* mParts 본문, 파일 모두 보낸다 ~~~~
**********************************************/
message.setContent(mParts);
// MIME 타입 설정
MailcapCommandMap MailcapCmdMap = (MailcapCommandMap) CommandMap.getDefaultCommandMap();
MailcapCmdMap.addMailcap("text/html;; x-java-content-handler=com.sun.mail.handlers.text_html");
MailcapCmdMap.addMailcap("text/xml;; x-java-content-handler=com.sun.mail.handlers.text_xml");
MailcapCmdMap.addMailcap("text/plain;; x-java-content-handler=com.sun.mail.handlers.text_plain");
MailcapCmdMap.addMailcap("multipart/*;; x-java-content-handler=com.sun.mail.handlers.multipart_mixed");
MailcapCmdMap.addMailcap("message/rfc822;; x-java-content-handler=com.sun.mail.handlers.message_rfc822");
CommandMap.setDefaultCommandMap(MailcapCmdMap);
// 메일 발송
Transport.send( message );
//System.out.println("메일을 발송 합니다 : "+sysdate);
}
개발시에는 네이버 SMTP로 다 만들었으면 회사로~~~
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.naver.com");
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.port", "587");
* 단순 메일<파일 첨부> 없을 때,
Multipart mParts = new MimeMultipart(); 객체는 파일 첨부일때만
MimeBodyPart
public void sendMessage2(String[] recipient_to,
String[] recipient_cc,
String[] recipient_bcc,
String subject,
String message,
String from,
String smtp_host_name,
String smtp_port) throws MessagingException {
Properties props = new Properties();
props.put("mail.smtp.host", smtp_host_name);
props.put("mail.smtp.port", smtp_port);
Session session = Session.getDefaultInstance(props);
MimeMessage msg = new MimeMessage(session);
InternetAddress addressFrom = new InternetAddress(from);
msg.setFrom(addressFrom);
// 받는 사람
//System.out.println("start addressTo");
InternetAddress[] addressTo = new InternetAddress[recipient_to.length];
for (int i = 0; i < recipient_to.length; i++) {
addressTo[i] = new InternetAddress(recipient_to[i]);
}
// 첨부
if(recipient_cc!=null){
InternetAddress[] addressCc = new InternetAddress[recipient_cc.length];
for (int i = 0; i < recipient_cc.length; i++) {
addressCc[i] = new InternetAddress(recipient_cc[i]);
}
msg.setRecipients(Message.RecipientType.CC, addressCc);
}
// 비밀참조
if(recipient_bcc!=null){
InternetAddress[] addressBcc = new InternetAddress[recipient_bcc.length];
for (int i = 0; i < recipient_bcc.length; i++) {
addressBcc[i] = new InternetAddress(recipient_bcc[i]);
}
msg.setRecipients(Message.RecipientType.BCC, addressBcc);
}
msg.setRecipients(Message.RecipientType.TO, addressTo);
msg.setSubject(subject, "UTF-8");
msg.setContent(message, "text/html;charset=UTF-8");
Transport.send(msg);
}
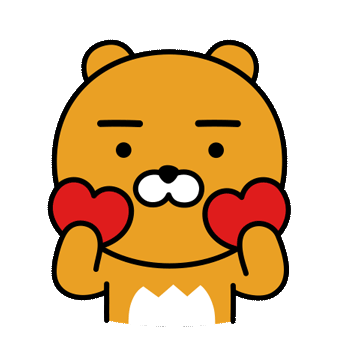
글이 유용했거나 도움이 되셨다면 공감 클릭 부탁합나다.
'슬기로운 자바 개발자 생활 > Java more' 카테고리의 다른 글
자바 LocalDateTime (0) | 2022.11.17 |
---|---|
jsonschema2pojo 사용하기 json데이터를 자바 클래스로 (0) | 2022.05.16 |
메서드 축출 기법(extract method) (0) | 2019.09.17 |
gson 사용 (0) | 2017.08.02 |
log4j java프로젝트 설정 (0) | 2017.08.02 |
댓글